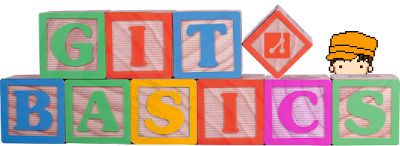
Here I'm going to run through the very basics of getting started with Git. Simply Git is used to store our server code. It is a LOT more powerful than that, but every needs to start with baby steps.
First step is to download/install the latest version of Git on your machine.
Now I am going to build on my node.js/coffee example.
Once you have the source running. I want you to point your terminal to your directory where you have the coffee source saved.
Terminal
Your path should now have "(Master)" at the end, but we now need to add our server code into the newly created repository.
git init
Terminal ~ This will stage all the files.
git add .
* You can think of staging like adding to a list of files that you are ready to commit.
Next we commit our staged file.
Terminal
git commit -m "First commit"
You should get something like the following:
[master (root-commit) 950b29b] First commit
1 file changed, 19 insertions(+)
create mode 100644 index.coffee
Let do a quick test to make sure all is good we our server
So far so good! but there is one small thing bugging me.. that console message when the server starts. lets make 2 small tweaks. We are going to print out the port number and make the port selection more dynamic by adding have an optional argument when starting the script to specify the port. Else check if there is a predefined port of servers to start on.
First we will read in the port number from the command line. For this we will need process.argv which is an array containing the command line arguments. The first element will be 'coffee', the second element will be the path to our file and the last element will be the port number. The second part is process.env.PORT this will try and pull a port number from the global environment variable.
Add at the top of the script
port = process.argv[2] port ?= process.env.PORT port ?= 80
Replace line 15 & 17 with the below !! don't forget the indentation !!
http.createServer(onRequest).listen (port) console.log ("Server on port #{port} has started.")
The changes above will read in a port value, If one can't be found 8888 will be set as a default. The second part sets the port number and will output the number when the server is started.
Terminal
coffee index.coffee 8889
You should get something like the following:
Server on port 8889 has started.
Now lets commit our newly modified file with the following two commands
Terminal ~ This will stage just the index.coffee file.
git add index.coffee
And that is for now.
git commit -m "the port number can be passed as a command line argument and the port number will be displayed on terminal"
No comments:
Post a Comment